This post is the next post of Part 1.
A series of posts is intended to see the basics of Python. In the previous post, the following contents were introduced.
- Variables
- Comment “#”
- Arithmetic operations
- Boolean
- Comparison operator
- List
In this post, we will learn the following contents.
- dictionary
- if statement
- for loop
- function
- instance
- class
Note) The sample code in this post is supposed to run on Jupyter Notebook.
dictionary
A dictionary stores pairs of “key” and “value”. We can access the “value” of an element by the “key”.
Here, let’s see the example of a dictionary, which stores fruit names and their numbers. A dictionary is defined by “{ }”. However, when we access the value of the dictionary, we use “[ ]” whose argument is its key.
dic_fruit = {"apple":1, "orange":3, "grape":5}
dic_fruit["grape"]
>> 5
When we pass a key that is NOT included in a dictionary, Python returns the error, where “nut” does NOT exist in the dictionary “dic_fruit”.
dic_fruit["nut"] # dic_fruit = {"apple":1, "orange":3, "grape":5}
>> ---------------------------------------------------------------------------
>> KeyError Traceback (most recent call last)
>> <ipython-input-11-2c89a279e528> in <module>()
>> ----> 1 dic_fruit["nut"]
>>
>> KeyError: 'nut'
It is easy to add a new element as follows.
dic_fruit["nut"] = 100
print(dic_fruit)
>> {'apple': 1, 'orange': 3, 'grape': 5, 'nut': 100}
The element of “nut” was added.
if statement
“if statement” is for a conditional statement, whether True or False. For example, if x is greater than zero, output “x > 0”. On the other hand, if x is less than zero, output “x < 0”. In Python, the above conditional statements are written as follows.
x = 5
if x > 0:
print( "x > 0" )
elif x < 0:
print( "x < 0" )
else:
print( "x = 0")
>> x > 0
In the above example, when x = 5, x > 0 is True. On the other hand, x < 0 is False. Note that we sometimes forget to add the colon “:”.
How about the input of “x = 0”? You can confirm the output “x = 0”, too.
The if statement branches according to the conditions. Python runs line by line from top to bottom lines.
----------------------------------------------
# condition1, condition2 are True or False.
if condition1:
Processing 1 # When, condition1 is True
elif condition2:
Processing 2 # When, condition2 is True
else:
Processing 3 # When, otherwise
----------------------------------------------
An indent is required at the beginning of the “processing” line. Python recognizes it as processing from whether an indent exists. Note that we sometimes forget to add the colon “:” at the ending of the “if condition”.
It is the same when the variable type is “str”.
x = "orange"
if x == "aplle":
print( "x is apple." )
else:
print( "x is NOT apple" )
>> x is NOT apple
By if statement, we can judge whether the element is included in the list. We use the “in” and “not in” operators.
words = ["orange", "grape", "peach"]
if "apple" in words:
print( "words includes apple." )
elif "apple" not in words:
print( "words NOT include apple." )
>> words NOT include apple.
The point is that the “if(elif)” part is executed when True, and the “else” part is executed when the above does not apply.
Python is an intuitive language so that we can easily confirm the conditions of the above example. From the following example, you can easily understand that if statement branches according to True or False.
"apple" in words # words = ["orange", "grape", "peach"]
>> False
"orange" in words # words = ["orange", "grape", "peach"]
>> True
x > 0 # x = 5
>> True
for loop
Repeat the same operation. We use “for loop” in such a case. Here, let’s see the example of printing numbers from 0 to 4.
for i in range(5):
print(i)
>> 0
>> 1
>> 2
>> 3
>> 4
In the “for loop”, the variable “i” changes in order from 0 to 4.
Note that, in Python, an index starts from 0. And, “range(5)” indicated the five consecutive integers starting from 0. Then, when you would like to print the values from 1 to 5, you should set them as follows.
for i in range(1, 6):
print(i)
>> 1
>> 2
>> 3
>> 4
>> 5
If you want to skip one, the code is below.
for i in range(1, 6, 2):
print(i)
>> 1
>> 3
>> 5
It is also possible to output the elements of the list storing the strings in order.
for name in words: # words = ["orange", "grape", "peach"]
print(name)
>> orange
>> grape
>> peach
function
A function is like a converter. For example, we can imagine the typical mathematical function, $f(x) = x^{2}$. The $f(x)$ converts the input $x$ into the output $x^{2}$, whose image is below. And, let’s see the code of this image.
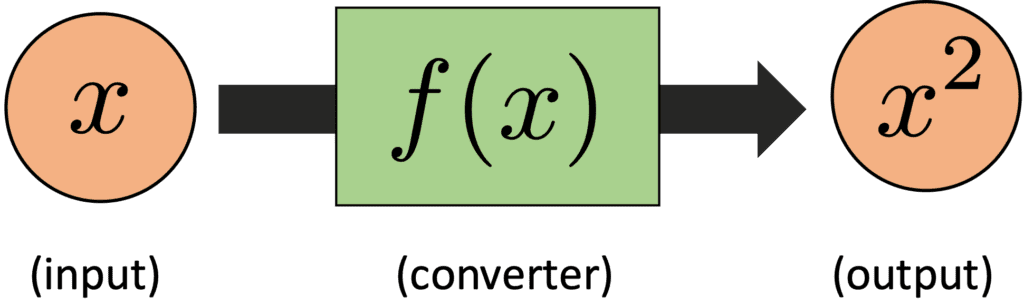
def func(x):
return x*x
print( func(5) )
>> 25
We defined the function by “def func(x):”, whose name is “func”. And, “x” is an argument, namely an input. And, “return x*x” means this function returns the value of $x^{2}$. Of course, the return value is not always necessary. In the following example, Python just executes the command written in the function “func_print()”.
def func_print():
print("This is a function")
print("without a returned value.")
func_print()
>> This is a function
>> without a returned value.
Why we use a function? This is because we can understand a code more readable. For example, if we write a set of codes as a function of $f(x)=x^{2}$, we can recognize it as mathematical calculus. In other words, by using a function, the blueprint of your code becomes clearer.
Let’s see the following example for the above description. Here, we consider converting $x$, which will vary from 1 to 10 in order, with the function $f(x)$. Note that $f(x)$ changes dependent on the range of $x$, whose conditional branching is shown in the figure below.
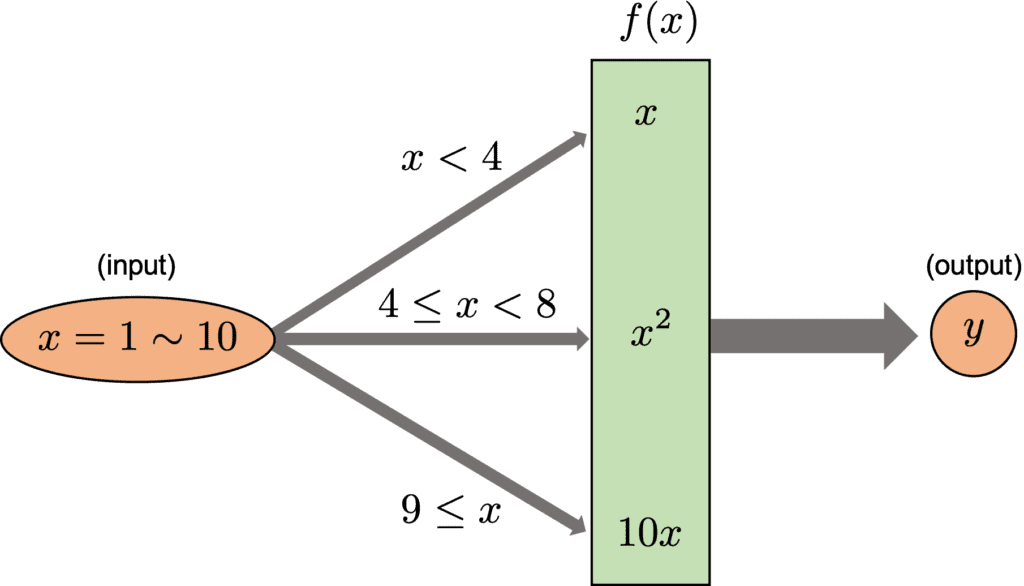
First, we will see the sample code without function.
for x in range(1, 11):
if x < 4: # x < 4
y = x
elif x < 8: # 4 <= x < 8
y = x*x
else:
y = 10*x # 9 <= x
print("x=", x, "was converted into y=", y)
>> x= 1 was converted into y= 1
>> x= 2 was converted into y= 2
>> x= 3 was converted into y= 3
>> x= 4 was converted into y= 16
>> x= 5 was converted into y= 25
>> x= 6 was converted into y= 36
>> x= 7 was converted into y= 49
>> x= 8 was converted into y= 80
>> x= 9 was converted into y= 90
>> x= 10 was converted into y= 100
Next, the sample code with function is below. We will see that the above code becomes more readable. Especially, it should be easier to understand what is going on in the for loop.
"""
y = f(x)
"""
def func(x):
if x < 4: # x < 4
return x
elif x < 8: # 4 <= x < 8
return x*x
else:
return 10*x # 9 <= x
for x in range(1, 11):
y = func(x)
print("x=", x, "was converted into y=", y)
>> x= 1 was converted into y= 1
>> x= 2 was converted into y= 2
>> x= 3 was converted into y= 3
>> x= 4 was converted into y= 16
>> x= 5 was converted into y= 25
>> x= 6 was converted into y= 36
>> x= 7 was converted into y= 49
>> x= 8 was converted into y= 80
>> x= 9 was converted into y= 90
>> x= 10 was converted into y= 100
In programming, it is very important to write a script with a combination of smaller functional codes. This will make your code easier to read and maintain. In other words, the clearer the blueprint of the code, the better the code.
One more thing you need to know about a function is a local or global variable. A local variable can be accessed just in a function. On the other hand, a global variable can be accessed anytime. Besides, the information of a variable defined in a function is lost after exiting the function.
Let’s understand this explanation through an example. Try to understand how the value of “a” changes with each step.
a = 5
##-- Step 1
print("Step 1: a =", a)
>> Step 1: a = 5
def func():
a = 10
##-- Step 2
print("Step 2: a =", a)
func()
>> Step 2: a = 10
##-- Step 3
print("Step 3: a =", a)
>> Step 3: a = 5
The above example indicates that the variables of “a” are different between outside and inside of the function “func()”.
Summary
Until here, the core syntax in Python has been introduced. In particular, the main topics (if statement, for loop, and function) are often used, so even beginners of Python should master them.
In the next post, we will try to learn more complex contents.