This post is the next post of Part 1 and 2. A series of posts is intended to see the basics of Python.
The following contents were already introduced in the previous posts, Part 1 and 2.
- variables
- comment
- arithmetic operations
- boolean
- comparison operator
- list
- dictionary
- if statement
- for loop
- function
In this Part 3, we will learn the following contents.
- object
- class
- instance
Note) The sample code in this post is supposed to run on Jupyter Notebook.
object
Python is an object-oriented programming language. An object-oriented style makes it possible to write a more readable and flexible code. Therefore, to understand the concept of an object is highly important.
However, as we have seen in Part 1 and 2, an object-oriented style doesn’t appear. But, it is just Python has hidden object orientation. But from here on, let’s take advantage of object-orientation and take it one step further. This will make your code more functional and maintainable.
Everything that Python deals with is an object. For example, variables, list, function, ..etc. An object is often likened to a thing. In Python, we call a concrete object an instance. The concept of an instance is unfamiliar to beginners. However, please note that it is needed especially when creating a machine learning model.
Example to understand object
Let’s imagine an object with some examples.
First, how about the variable $x$, whose value is $1$.
x = 1
x
>> 1
You may be thinking that $x$ is a variable with the value of $1$. Or, $x$ equals $1$. However, recall the following fact.
type(x)
>> int
Actually, the variable $x$ has a value of $1$ and the information of data type of “int“. We don’t usually think about the above. However, $x$ is a variable object that has value and data-type information.
We’re not aware of it because we just gave $x$ a value of $1$. But, in behind, Python also gives variable attribute information.
Next, let’s see another example of list.
a = [1, 2, 3]
type(a)
>> list
The list $a$ has the values of [1, 2, 3] and the information of list. Here, please recall that we can add new element by the append() method.
a.append(10)
a
>> [1, 2, 3, 10]
When be aware of object, the list object $a$ has the method append() and we called it by $a$.append(). In other words, the append() method was originally included in the list object $a$. The list object has values, information of data type, and functions.
Short summary of object
Could you have imagined an object from the above example? An object is a thing including values, information, and functions. Note that variables, lists, functions, etc. are objects that Python has as standard. We were unknowingly calling and using it.
From here, you will create your own objects with a next topic called “class”. Especially, when creating a machine learning model, we need to create our original object by class(). This is due to designing machine learning models by giving the objects model structure, training, and predictive functions.
class
Here, let’s create our own object by class. The sample code is below. We define a class by “class (class name)”. In the following, we created the class “MyClass()”. And, “__init__()” is for initializing an argument $x$ when we create an instance from the class. Although it is unfamiliar to beginners, a function in class must receive the own argument “self”, which is just an object itself. Then, each function in the class also receives “self”, making it possible to use the variables(self.x) and the functions(func1, func2, func3).
class MyClass():
def __init__(self, x):
self.x = x
# f(x) = x
def func1(self):
return self.x
# f(x) = x^2
def func2(self):
return self.x*self.x
# f(x) = 10*x
def func3(self):
return 10*self.x
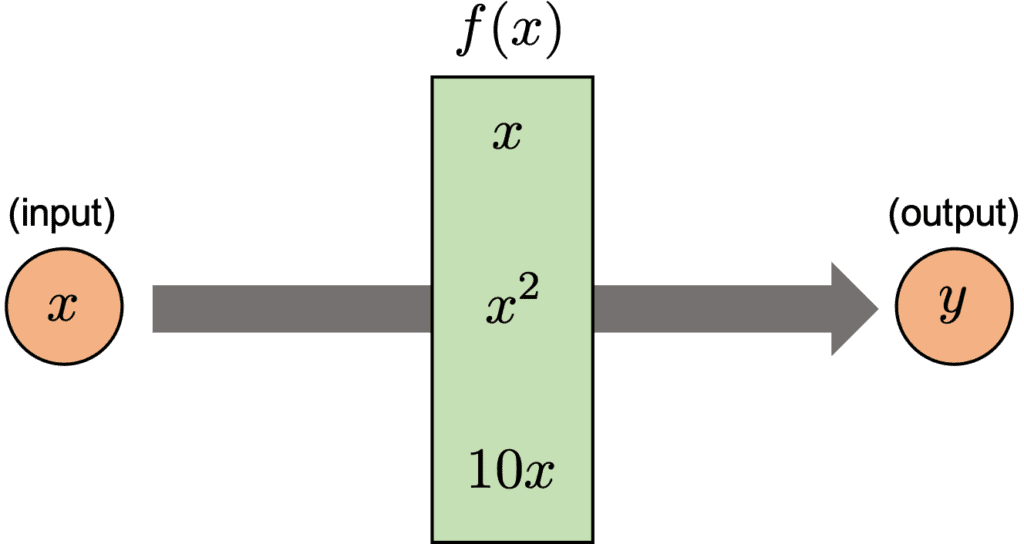
instance
An instance is a thing created from a class. Here, let’s create an instance with name of “instance” from the class “MyClass()”.
x = 5
instance = MyClass(x)
This instance has three functions(func1, func2, func3) defined in “MyClass()”. These functions can be called in the form of methods.
instance.func1() # f(x) = x
>> 5
instance.func2() # f(x) = x^2
>> 25
instance.func3() # f(x) = 10*x
>> 50
Here, the “__call__()” method is introduced. This method is called without the form of “.method()”. Let’s take the following example. The shaded area is where “__call__()” was added.
class MyClass_updated():
def __init__(self, x):
self.x = x
def __call__(self):
if self.x < 4:
return self.func1()
elif self.x < 8:
return self.func2()
else:
return self.func3()
def func1(self):
return self.x
def func2(self):
return self.x*self.x
def func3(self):
return 10*self.x
Then, create an instance from the class “MyClass_updated()” and call the instance. The point is that the “__call__()” is called at “instance()”.
x = 5
instance = MyClass_updated(x)
instance() # __call__() is called
>> 25
At this point, we can convert $x$, which will vary from $1$ to $10$ in order, with the function $f(x)$. Note that $f(x)$ changes dependent on the range of $x$, whose conditional branching is shown in the figure below.
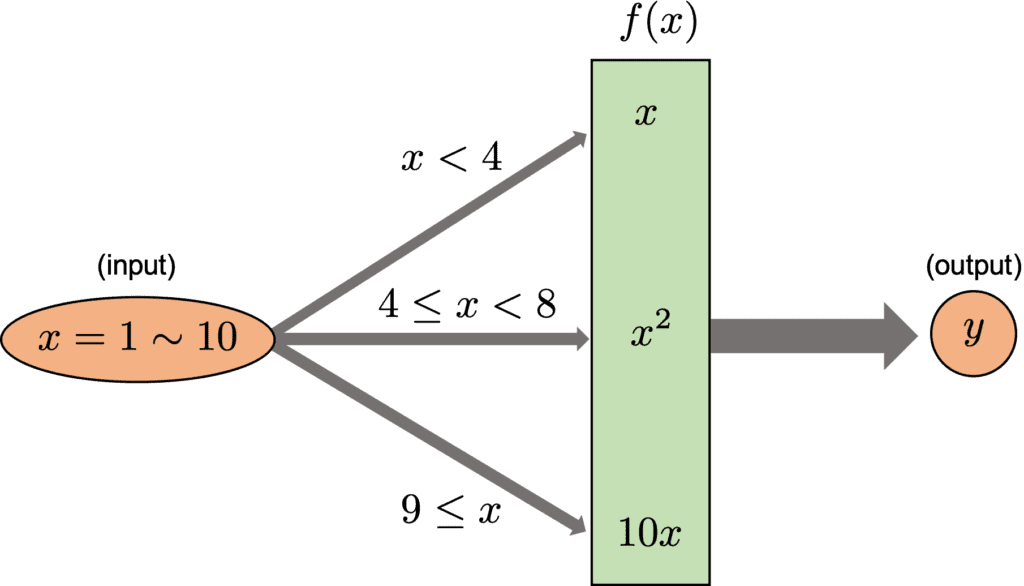
for x in range(1, 11):
instance = MyClass_updated(x)
print( instance() ) # __call__() is called
>> 1
>> 2
>> 3
>> 16
>> 25
>> 36
>> 49
>> 80
>> 90
>> 100
Summary
We have seen an object, class, and instance in Python. These topic may be unfamiliar to beginners. However, these are important especially for a data scientist. The basics of Python are covered in the series of posts of Part 1 – 3.
The next step is to learn the external Python library, such as NumPy, Pandas, and scikit-learn, for data science and machine learning. By calling these libraries from Python, you can take advantage of various functions. For example, NumPy makes it easier to perform numerical calculations. Pandas is useful for the treatment of table data. And, we can create a machine-learning model with low-codes by using scikit-learn.
Note that what you call from an external library is just a class someone created. You have already basic knowledge. And you can also create your own external library.
The author hopes this blog helps readers a little.